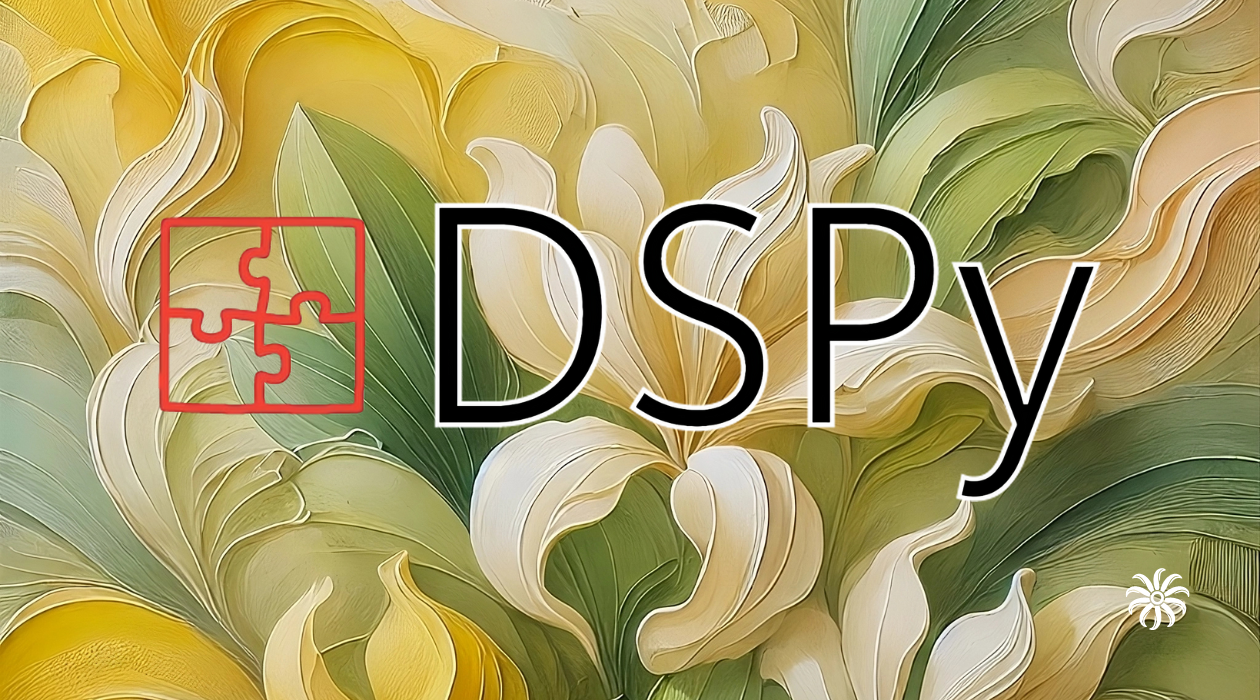
DSPy marks a revolutionary shift in how developers build applications powered by Large Language Models (LLMs). Instead of wrestling with brittle prompts and time-consuming manual optimizations, DSPy provides a programmatic framework that makes LLM development more modular, maintainable, and production-ready.
In this guide, you’ll learn:
- Why traditional prompt engineering struggles with complexity
- How DSPy’s programmatic abstractions transform LLM development
- Essential principles and patterns for creating reliable AI systems
- When to Use DSPy vs. Traditional Prompt Engineering
- Best practices for building production-scale DSPy applications
By the end, you’ll understand why DSPy is fast becoming the go-to choice for teams creating sophisticated LLM-driven solutions.
1. Introduction to DSPy
1.1 The Evolution from Prompting to Programming
Most prompt engineers have experienced the frustration of painstakingly crafting prompts. For each task, the number of possible prompts is infinite, making manual prompt engineering an exhausting and time-consuming process. The optimal prompt remains elusive - even when you think you've found the perfect one, a slight tweak can break everything.
The Limitations of Traditional Prompting
- Brittle Solutions: Tiny changes can derail LLM responses.
- Poor Maintainability: Long, tangled prompts are tough to debug.
- Limited Composability: Combining multiple prompts in a structured way is cumbersome.
- Optimization: Fine-tuning prompts by hand is slow and error-prone.
- Lack of Systematic Testing: Hard to evaluate effectiveness across diverse scenarios.
# Traditional approach - brittle and hard to maintain
prompt = """Given a sentence, determine if it's positive or negative.
Examples:
'I love this!' -> positive
'This is terrible' -> negative
Now analyze: '{input_text}'"""
The DSPy Paradigm Shift
DSPy flips the script by letting you define LLM interactions as “programs” instead of manual prompts:
Declarative Programming
# DSPy approach - clean, maintainable, and optimizable class SentimentAnalyzer(dspy.Signature): """Analyze the sentiment of a sentence.""" text: str = dspy.InputField() sentiment: Literal['positive', 'negative'] = dspy.OutputField()
- Focus on what the LLM should do, not how.
- Use Python classes with typed fields.
- Structure everything for clarity and maintainability.
Modular Design
class ReviewAnalyzer(dspy.Module): def __init__(self): self.sentiment = dspy.ChainOfThought(SentimentAnalyzer) self.summarize = dspy.ChainOfThought('review -> key_points: list[str]')
- Break tasks into composable, reusable components.
- Easily swap modules without refactoring the entire system.
Systematic Optimization
optimizer = dspy.BootstrapFewShot(metric=accuracy_metric) optimized_analyzer = optimizer.compile(sentiment_analyzer, trainset=examples)
- Automate prompt improvements with training data.
- Leverage machine learning principles for better results.
1.2 Core DSPy Philosophy
DSPy’s philosophy rests on three pillars: Declarative Programming, Separation of Concerns, and Building Maintainable Systems.
Declarative Programming with LLMs
- Define Signatures using structured fields and type hints.
- Use Modules to encapsulate logic.
Separation of Concerns
- Keep Signatures (input/output) separate from the underlying prompt logic.
- Let DSPy handle LLM interactions, so your business logic remains in standard Python code.
Building Maintainable Systems
- Enforce Testability with clear metrics and test sets.
- Encourage Modularity for easy component reuse.
- Support Optimization for continuous performance gains.
1.3 DSPy vs Other Frameworks
Various frameworks have emerged for LLM development, but DSPy stands out for its automation and systematic optimization.
Feature | DSPy | LangChain | LlamaIndex |
---|---|---|---|
Core Philosophy | Programmatic LLM control with auto-optimization | Prompt/chain orchestration | Document-centric data structuring |
Prompt Management | Automatic generation & refinement through compilers | Templates and manual tuning | Template-based approach |
Optimization | Built-in, data-driven optimization of prompts and module weights | Manual chain adjustments | Manual retriever/prompt tuning |
Best Suited For | Production systems needing reliability and iterative improvement | Rapid workflow prototyping | Knowledge-base and document retrieval |
2. Getting Started with DSPy
2.1 Setup and Configuration
DSPy is designed to be LLM-agnostic, thanks to its integration with LiteLLM. You can seamlessly switch between LLM providers or even self-hosted models without significant refactoring.
Install DSPy
pip install dspy-ai python-dotenv
Environment Configuration
# .env file WATSONX_URL=your_watsonx_url WATSONX_APIKEY=your_api_key WATSONX_PROJECT_ID=your_project_id
LLM Setup
import dspy import os from dotenv import load_dotenv load_dotenv() WATSONX_URL = os.getenv("WATSONX_URL") WATSONX_APIKEY = os.getenv("WATSONX_APIKEY") WATSONX_PROJECT_ID = os.getenv("WATSONX_PROJECT_ID") lm = dspy.LM( "watsonx/meta-llama/llama-3-8b-instruct", project_id=WATSONX_PROJECT_ID, ) dspy.configure(lm=lm, trace=[], temperature=0.7)
2.2 Your First DSPy Program
Let’s make a simple QA system to get a feel for DSPy’s development flow.
Define the Signature
class BasicQA(dspy.Signature): """Answer questions with short factoid answers.""" question = dspy.InputField() answer = dspy.OutputField(desc="often between 1 and 5 words")
Create and Use the Predictor
generate_answer = dspy.Predict(BasicQA) test_question = "What country was the winner of the Nobel Prize in Literature in 2006 from?" prediction = generate_answer(question=test_question) print(f"Answer: {prediction.answer}")
2.3 Working with Data in DSPy
DSPy uses Example
objects to manage training, validation, and testing data consistently.
qa_pair = dspy.Example(
question="Who won the Nobel Prize in Literature in 2006?",
answer="Orhan Pamuk from Turkey"
).with_inputs("question")
trainset = [
dspy.Example(question="What is the capital of France?", answer="Paris").with_inputs("question"),
# More examples...
]
2.4 Best Practices for Dataset Creation
- Diverse Examples: Include a range of topics and question types.
- High-Quality Data: Keep answers accurate and formatting consistent.
- Organized Splits: Separate training, dev, and test sets.
- Data Augmentation: Consider rephrasing or generating synthetic variations for broader coverage.
3. Core DSPy Components
3.1 Signatures: The Building Blocks
Signatures define how your LLM program interacts with the outside world (input/output). You can use simple inline formats or full-fledged classes:
basic_qa = dspy.Predict("question: str -> answer: str")
Or for more complexity:
from typing import Literal
class EmotionClassifier(dspy.Signature):
"""Classify emotion and confidence."""
text = dspy.InputField()
emotion: Literal['joy', 'sadness', 'anger', 'fear', 'surprise'] = dspy.OutputField()
confidence: float = dspy.OutputField()
3.2 Modules: DSPy's Workhorses
Modules implement various prompting and reasoning strategies:
- Predict – Direct single-step predictions.
- ChainOfThought – Step-by-step reasoning for more complex tasks.
- RAG – Retrieval-Augmented Generation for grounding outputs in external knowledge.
- ReAct – Advanced pattern combining reasoning with tool usage.
Example: a ReAct agent that can search Wikipedia or perform calculations:
def search_wikipedia(query: str) -> list[str]:
return ["Sample search result"]
def calculate(expression: str) -> float:
return eval(expression)
react_agent = dspy.ReAct(
"question -> answer",
tools=[search_wikipedia, calculate]
)
result = react_agent(question="What is the population of France divided by 2?")
3.3 Metrics
DSPy uses metrics to quantify performance—like exact match accuracy or more nuanced custom checks:
from dspy.evaluate.metrics import answer_exact_match
def validate_answer(example, pred, trace=None):
return example.answer.lower() == pred.answer.lower()
Use the Evaluate
class to run tests:
from dspy.evaluate import Evaluate
evaluator = Evaluate(devset=dev_examples, display_progress=True)
results = evaluator(predictor, metric=validate_answer)
3.4 Optimization Techniques
To automatically tune prompts and modules, DSPy provides powerful optimizers:
- Zero-Shot MIPROv2: Optimizes instructions with no few-shot examples.
- BootstrapFewShot: Best for small training datasets (5-50 examples).
- MIPROv2: Great for larger datasets and deeper optimization.
optimizer = dspy.BootstrapFewShot(metric=accuracy_metric, max_bootstrapped_demos=4)
optimized_predictor = optimizer.compile(student=predictor, trainset=train_examples)
Optimizer | Data Size | Optimization Target | Time | Use Case |
---|---|---|---|---|
Zero-shot MIPROv2 | 50+ examples | Instructions only | Hours | Minimal prompt length needed |
BootstrapFewShot | 5-50 examples | Few-shot examples | Minutes | Quick iteration with small data |
MIPROv2 | 50+ examples | Instructions & examples | Hours | Complex tasks, thorough optimization |
4. When to Use DSPy vs. Traditional Prompt Engineering
Choosing between DSPy and traditional prompt engineering depends largely on the complexity and scalability requirements of your AI application. Like other prompt optimization tools, DSPy aims to automate the prompt engineering workflow. However, it's crucial to understand both the advantages and potential pitfalls of such automation.
4.1 Understanding the Cost-Benefit Tradeoff
Before diving into DSPy, consider these important factors:
API Costs:
- DSPy's optimization process generates multiple API calls behind the scenes
- Each prompt variation may require multiple calls: generation, validation, and scoring
- Complex chains can exponentially increase API usage
Development Overhead:
- Initial setup time for DSPy infrastructure
- Learning curve for the programmatic approach
- Time saved in long-term maintenance and optimization
Quality vs. Speed Tradeoff:
- Traditional prompting: Faster to start, but more time-consuming to maintain
- DSPy: Higher upfront investment, but better long-term scalability
Tool Developer Errors:
- Prompt template mismatches between models
4.2 When to Use Traditional Prompt Engineering
While DSPy shines in complex scenarios, traditional prompt engineering is still valuable in certain contexts:
Simple and Direct Tasks
- Quick translations or basic information retrieval
- Single-response generation without complex logic
prompt = "Translate the following English sentence to French: 'Hello, how are you?'"
Rapid Prototyping
- Early-stage development and experimentation
- Quick validation of ideas before full implementation
Low Complexity Applications
- Personal projects and small-scale applications
- Cases where setup overhead outweighs benefits
Below is a more concise, story-like version of Section 5. It preserves the key ideas but keeps language simple and direct.
5. Best Practices for Building Production-Scale DSPy Applications
Imagine you’ve just guided your DSPy prototype through testing, and it’s working smoothly. Now, the real test begins: running it in production, handling live user data, and staying within budget. These best practices will help your DSPy application evolve from an exciting demo into a stable, cost-effective system.
5.1 Designing for Reliability and Maintenance
Keep Signatures Clear
Each Signature acts like a contract. Document inputs, outputs, and edge cases so issues can’t hide in the details.Modularize Your Design
Treat each module as a self-contained puzzle piece. If one piece fails, you can fix or swap it without breaking everything else.Test Thoroughly
From normal scenarios to edge cases, make testing a habit. DSPy’s evaluation tools can catch small flaws before they snowball.Automate Optimization
Use DSPy’s built-in optimizers regularly to refine prompts. This helps you stay ahead of shifting data and user demands.Version and Document Everything
Every tweak to your Signatures, prompts, or datasets should be recorded. Clear documentation saves time when troubleshooting or rolling back changes.
5.2 Managing Costs and Complexity
Start Simple
Begin with straightforward modules (e.g.,Predict
) and a modest dataset. Add complexity only when needed.Optimize Wisely
Each optimization run triggers extra API calls. Focus first on the biggest performance gaps to manage costs effectively.Regularly Check Generated Prompts
Automated prompts can become repetitive or bloated over time. Trim them to keep responses efficient.
5.3 Iterating Toward Production Readiness
Establish a Baseline
Launch a minimal viable product (MVP) and track key metrics. This baseline guides your future upgrades.Monitor and Validate
Watch logs for unusual responses or spikes in costs. When issues arise, trace them back to specific modules or prompts.Scale Gradually
Introduce advanced DSPy features one step at a time. Validate each change so you know exactly what boosted performance—or caused a slip.
Summary
- Programming Layer
- Task definition and initial pipeline design
- Signatures define input/output behavior
- Modules implement LM programs
Evaluation Layer
- Metrics assess performance
- Development set provides test examples
- Systematic evaluation guides improvements
Optimization Layer
- Training set provides examples for optimization
- Optimizer tunes prompts and weights
- Creates production-ready LM programs
The flow shows how these layers work together in a systematic way: from defining the task and implementing the logic, through evaluation and testing, to optimization with training data and deployment.
Conclusion
Through this guide, we’ve explored how DSPy transforms LLM development from manual prompt engineering into a structured, programmatic practice. We learned the limitations of traditional prompting—its brittleness, poor maintainability, and lack of systematic testing—and how DSPy addresses these challenges with declarative programming, modular design, and automated optimization.
We also saw practical examples of DSPy in action: from creating clear Signatures and reusable modules to leveraging built-in tools for evaluation and automated optimization. Along the way, best practices like modularization, testing, and iterative optimization revealed how DSPy simplifies building robust, production-ready AI systems.
By adopting DSPy, you’re not just creating better prompts—you’re crafting AI applications that are scalable, maintainable, and ready to evolve with future demands.
Ready to dive deeper? Check out the DSPy documentation for more recipes, advanced modules, and a growing developer community.
References
DSPy Documentation https://dspy.ai/
Huyen, C. AI Engineering: Building Applications with Foundation Models, O'Reilly Media, 2024 https://learning.oreilly.com/library/view/ai-engineering/9781098166298/
Prompting with DSPy: A New Approach https://www.digitalocean.com/community/tutorials/prompting-with-dspy
DSPy: Compiling Declarative Language Model Calls into Self-Improving Pipelines https://arxiv.org/abs/2310.03714